3 June 2024
05 Min. Read
What is Mockito Mocks: Best Practices and Examples
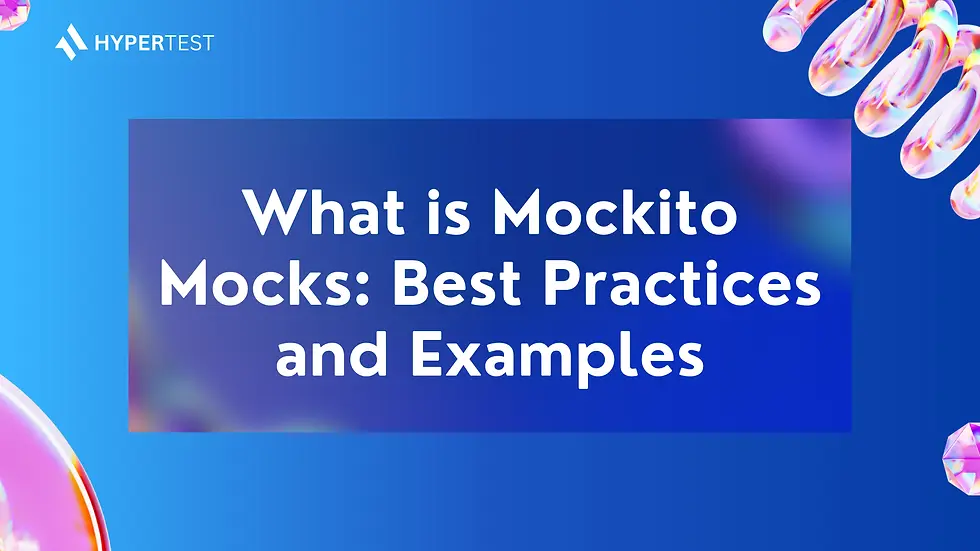
Fast Facts
Get a quick overview of this blog
Mockito mocks isolate your code for unit testing, but require manual setup and maintenance.
Use Mockito for well-defined interactions, consider HyperTest for complex scenarios or legacy code.
Focus on mocking external dependencies, not internal implementation details.
Keep mock behavior clear and concise for better test readability.
Hey everyone, let's talk about Mockito mocks!
As engineers, we all know the importance of unit testing. But what happens when your code relies on external dependencies, like databases or services? Testing these dependencies directly can be cumbersome and unreliable. That's where Mockito mocks come in!
Why Mocks?
Imagine testing a class that interacts with a database. A real database call can be slow and unpredictable for testing. With Mockito, we can create a mock database that behaves exactly how we need it to, making our tests faster, more reliable, and easier to maintain.
What are Mockito Mocks?
Think of Mockito mocks as stand-ins for real objects. They mimic the behavior of those objects, allowing you to control how they respond to method calls during your tests. This isolation empowers you to:
Focus on the code you're writing: No more worrying about external dependencies slowing down or interfering with your tests.
Predict behavior: You define how the mock behaves, eliminating surprises and ensuring tests target specific functionalities.
Simulate different scenarios: Easily change mock behavior between tests to explore various edge cases and error conditions.
Imagine a fake collaborator for your unit test. You define how it behaves, and your code interacts with it as usual. Mockito lets you create these "mock objects" that mimic real dependencies but under your control.
Here's a simple flowchart to visualize the concept:

Why Use Mockito Mocks?
Isolation: Test your code in isolation from external dependencies, leading to faster and more reliable tests.
Control: Define how mock objects behave, ensuring consistent test environments.
Flexibility: Easily change mock behavior for different test scenarios.
Getting Started with Mockito Mocks
1.Add Mockito to your project: Check your build system's documentation for including Mockito as a dependency.
2.Create a Mock Object: Use the mock() method from Mockito to create a mock object for your dependency:
// Import Mockito
import org.mockito.Mockito;
// Example: Mocked Database
Database mockDatabase = Mockito.mock(Database.class);
3. Define Mock Behavior: Use when() and thenReturn() to specify how the mock object responds to method calls:
// Mock database to return a specific value
when(mockDatabase.getUser(1)).thenReturn(new User("John Doe", "john.doe@amazon.com"));
Best Practices for Using Mockito Mocks
Focus on Behavior, Not Implementation: Don't mock internal implementation details. Focus on how the mock object should behave when interacted with.
Use Argument Matchers: For flexible matching of method arguments, use Mockito's argument matchers like any() or eq().
Verify Interactions: After your test, use Mockito's verification methods like verify() to ensure your code interacted with the mock object as expected.
Clean Up: Mockito mocks are typically created within a test method. This ensures a clean slate for each test run.
Putting it all together: Testing a User Service
Let's see how Mockito mocks can be used to test a user service that retrieves user data from a database:
public class UserService {
private final Database database;
public UserService(Database database) {
this.database = database;
}
public User getUser(int userId) {
return database.getUser(userId);
}
}
// Test for UserService
@Test
public void testGetUser_ValidId() {
// Mock the database
Database mockDatabase = Mockito.mock(Database.class);
when(mockDatabase.getUser(1)).thenReturn(new User("Jane Doe", "jane.doe@amazon.com"));
// Create the user service with the mock
UserService userService = new UserService(mockDatabase);
// Call the service method
User user = userService.getUser(1);
// Verify interactions and assert results
Mockito.verify(mockDatabase).getUser(1);
assertEquals("Jane Doe", user.getName());
}
But do you really need to do manual effort?
While Mockito mocks offer a powerful solution, it is not without its drawbacks:
Final, Static, and Private Methods:
Mockito cannot mock final methods, static methods, or methods declared as private within the class you want to mock. This can be a challenge if your code relies heavily on these methods. There are workarounds using third-party libraries like PowerMock, but they can introduce complexity.
Manual Effort:
Mock Setup and Maintenance: Creating mocks, defining their behavior for various scenarios, and verifying their interactions during tests can be time-consuming, especially for complex dependencies. As your code evolves, mocks might need to be updated to reflect changes, adding to the maintenance burden.
Limited Error Handling:
Simulating Real-World Errors: Mocks might not accurately simulate all the potential error conditions that can occur with real external systems. This can lead to incomplete test coverage if you don't carefully consider edge cases.
These limitations suggest that Mockito mocks are not complete without the mock object. For complex scenarios or when mocking final/static/private methods becomes a hurdle, consider alternative like HyperTest.
Mockito Vs HyperTest
HyperTest is a smart auto-mock generation testing tool that enables you to record real interactions with external systems and replay them during integration tests. This eliminates the need for manual mocking and simplifies the testing process, especially for integrations with external APIs or legacy code.
Feature | Mockito | HyperTest |
Mocking Style | In-memory mocking | Interaction recording & replay |
Suitable for | Well-defined, isolated interactions | Complex interactions, external APIs, legacy code |
Manual Effort | High (mock creation, behavior definition) | Lower (record interactions, less maintenance) |
Maintenance | Can be high as code and mocks evolve | Lower as replays capture real interactions |
+-------------------+
| Does your code |
| rely on external |
| dependencies? |
+-------------------+
|
Yes
v
+-------------------+
| Is the interaction |
| simple and well- |
| defined? |
+-------------------+
|
Yes (Mock)
v
+-------------------+
| Use Mockito mocks |
| to isolate your |
| code and test in |
| isolation. |
+-------------------+
|
No (Complex)
v
+-------------------+
| Consider using |
| HyperTest to |
| record and replay |
| real interactions |
+-------------------+
Conclusion
Mockito mocks are a powerful tool for writing reliable unit tests. By isolating your code and controlling dependencies, you can ensure your code functions as expected’.
Remember, clear and concise tests are essential for maintaining a healthy codebase. So, embrace Mockito mocks and write better tests, faster!
Related to Integration Testing