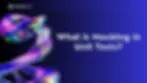
Fast Facts
Get a quick overview of this blog
Conducting Mocking Workshops: Hold regular sessions to discuss and practice mocking techniques.
Code Reviews: Emphasize the use of mocks during code reviews to ensure best practices are followed.
Documentation: Create and maintain documentation on how to use mocks in your projects.
Introduction to Unit Testing
Unit testing is a fundamental practice in software development where individual units or components of the software are tested in isolation. The goal is to validate that each unit functions correctly. A unit is typically a single function, method, or class. Unit tests help identify issues early in the development process, leading to more robust and reliable software.
What is Mocking?
Mocking is a technique used in unit testing to replace real objects with mock objects. These mock objects simulate the behavior of real objects, allowing the test to focus on the functionality of the unit being tested. Mocking is particularly useful when the real objects are complex, slow, or have undesirable side effects (e.g., making network requests, accessing a database, or depending on external services).
Why Use Mocking?
Isolation:
By mocking dependencies, you can test units in isolation without interference from other parts of the system.
Speed:
Mocking eliminates the need for slow operations such as database access or network calls, making tests faster.
Control:
Mock objects can be configured to return specific values or throw exceptions, allowing you to test different scenarios and edge cases.
Reliability:
Tests become more predictable as they don't depend on external systems that might be unreliable or unavailable.

How to Implement Mocking?
Let's break down the process of mocking with an example. Consider a service that fetches user data from a remote API.
Step-by-Step Illustration:
a. Define the Real Service:
class UserService {
async fetchUserData(userId) {
const response = await fetch(`https://api.example.com/users/${userId}`);
return response.json();
}
}
b. Write a Unit Test Without Mocking:
const userService = new UserService();
test('fetchUserData returns user data', async () => {
const data = await userService.fetchUserData(1);
expect(data).toHaveProperty('id', 1);
});
This test makes an actual network call, which can be slow and unreliable.
c. Introduce Mocking:
To mock the fetchUserData method, we'll use a mocking framework like Jest.
const fetch = require('node-fetch');
jest.mock('node-fetch');
const { Response } = jest.requireActual('node-fetch');
const userService = new UserService();
test('fetchUserData returns user data', async () => {
const mockData = { id: 1, name: 'John Doe' };
fetch.mockResolvedValue(new Response(JSON.stringify(mockData)));
const data = await userService.fetchUserData(1);
expect(data).toEqual(mockData);
});
Here, fetch is mocked to return a predefined response, ensuring the test is fast and reliable.
Mocking in Unit Tests
+-------------------+ +---------------------+
| Test Runner | ----> | Unit Under Test |
+-------------------+ +---------------------+
|
v
+-------------------+ +---------------------+
| Mock Object | <---- | Dependency |
+-------------------+ +---------------------+
1. The test runner initiates the test.
2. The unit under test (e.g., fetchUserData method) is executed.
3. Instead of interacting with the real dependency (e.g., a remote API), the unit interacts with a mock object.
4. The mock object returns predefined responses, allowing the test to proceed without involving the real dependency.
Use Cases for Mocking
Testing Network Requests:
Mocking is essential for testing functions that make network requests. It allows you to simulate different responses and test how your code handles them.
Database Operations:
Mocking database interactions ensures tests run quickly and without requiring a real database setup.
External Services:
When your code interacts with external services (e.g., payment gateways, authentication providers), mocks can simulate these services.
Complex Dependencies:
For units that depend on complex systems (e.g., large data structures, multi-step processes), mocks simplify the testing process.
Best Practices for Mocking
Keep It Simple:
Only mock what is necessary. Over-mocking can make tests hard to understand and maintain.
Use Mocking Libraries:
Leverage libraries like Jest, Mockito, or Sinon to streamline the mocking process.
Verify Interactions:
Ensure that your tests verify how the unit interacts with the mock objects (e.g., method calls, arguments).
Reset Mocks:
Reset or clear mock states between tests to prevent interference and ensure test isolation.
Problems with Mocking
While mocking is a powerful tool in unit testing, it comes with its own set of challenges and limitations:
1. Over-Mocking:
Problem:
Over-reliance on mocking can lead to tests that are tightly coupled to the implementation details of the code. This makes refactoring difficult, as changes to the internal workings of the code can cause a large number of tests to fail, even if the external behavior remains correct.
If every dependency in a method is mocked, any change in how these dependencies interact can break the tests, even if the overall functionality is unchanged.
2. Complexity:
Problem:
Mocking complex dependencies can become cumbersome and difficult to manage, especially when dealing with large systems. Setting up mocks for various scenarios can result in verbose and hard-to-maintain test code.
A service that relies on multiple external APIs may require extensive mock configurations, which can obscure the intent of the test and make it harder to understand.
3. False Sense of Security:
Problem:
Tests that rely heavily on mocks can give a false sense of security. They may pass because the mocks are configured to behave in a certain way, but this does not guarantee that the system will work correctly in a real environment.
Mocking a database interaction to always return a successful result does not test how the system behaves with real database errors or performance issues.
4. Maintenance Overhead:
Problem:
Keeping mock configurations up-to-date with the actual dependencies can be a significant maintenance burden. As the system evolves, the mocks need to be updated to reflect changes in the dependencies.
When a third-party API changes, all the mocks that simulate interactions with that API need to be updated, which can be time-consuming and error-prone.
How HyperTest is Solving Mocking Problems?
HyperTest, our integration testing tool, addresses these problems by providing a more efficient and effective approach to testing. Here’s how HyperTest solves the common problems associated with mocking:
Eliminates Manual Mocking:
HyperTest automatically mocks external dependencies like databases, queues, and APIs, saving development time and effort.
Adapts to Changes:
HyperTest refreshes mocks automatically when dependency behavior changes, preventing test flakiness and ensuring reliable results.
Realistic Interactions:
HyperTest analyzes captured traffic to generate intelligent mocks that accurately reflect real-world behavior, leading to more effective testing.
Improved Test Maintainability:
By removing the need for manual mocking code, HyperTest simplifies test maintenance and reduces the risk of regressions.
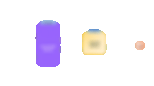
Conclusion
While mocking remains a valuable unit testing technique for isolating components, it can become cumbersome for complex integration testing. Here's where HyperTest steps in.
HyperTest automates mocking for integration tests, eliminating manual effort and keeping pace with evolving dependencies. It intelligently refreshes mocks as behavior changes, ensuring reliable and deterministic test results. This frees up development resources and streamlines the testing process, allowing teams to focus on core functionalities.
In essence, HyperTest complements your mocking strategy by tackling the limitations in integration testing, ultimately contributing to more robust and maintainable software.
or if you wish to explore more about it first, here’s the right place to go to.
Related to Integration Testing