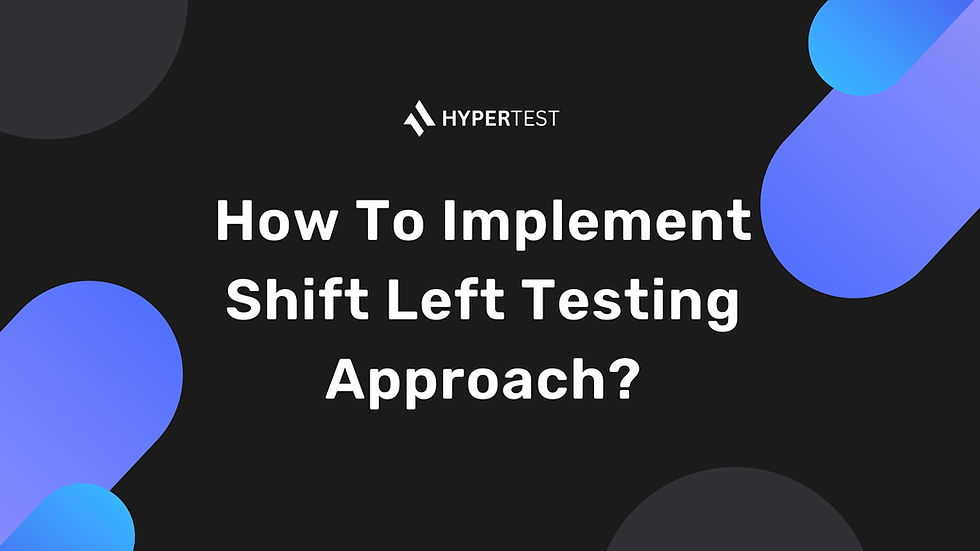
Fast Facts
Get a quick overview of this blog
Learn why shift-left testing is gaining popularity lately
Get to know about details on how shift left testing approach seamlessly fits into the agile development
Get insights on how to implement it for faster and robust releases
See how HyperTest can help your SDLC cycle in shifting towards left and catching bugs beforehand
In the rapidly evolving world of software development, the quest for efficiency and quality has led to the adoption of various methodologies aimed at enhancing the software development lifecycle (SDLC). Among these, Shift Left Testing has emerged as a pivotal approach, fundamentally altering how testing is integrated within the development process.
This method advocates for initiating testing activities earlier in the SDLC, thereby shifting the testing phase to the "left" on the project timeline. The essence of Shift Left Testing lies in its preventative philosophy, aiming to detect and address defects in the early stages of software development rather than at the end.
Shift Left Testing approach represents not just a methodology but a cultural transformation towards embracing quality as a foundational aspect of software development. It is a testament to the industry's ongoing evolution, reflecting a deeper understanding of the dynamics between speed, quality, and efficiency in creating software that meets and exceeds the demands of users and stakeholders alike.
The Systems Sciences Institute at IBM revealed that addressing a bug discovered in the implementation phase is six times more expensive than rectifying one identified during the design phase.
Additionally, IBM indicates that the expense of fixing bugs detected in the testing phase could be fifteen times higher than the cost of addressing those identified during the design phase.
Importance of Shift Left Testing
The Shift Left Testing approach is gaining traction within the software development community, not merely as a trend but as a significant evolution in enhancing the quality and reliability of software products. Here are some of the key advantages that make Shift Left Testing particularly appealing to everyone looking to release faster and save costly bug detection at later stages:
➡️Early Bug Detection and Resolution
At the heart of Shift Left Testing is the principle of early detection and resolution of bugs. By testing early and often in the development cycle, bugs are identified before they can evolve into more complex and costly problems.
This early intervention is not just a cost-saving measure; it significantly improves the stability and reliability of the software. For devs, this means less time spent backtracking to resolve issues in code that was considered complete, allowing for a more streamlined and efficient development process.
➡️Enhanced Collaboration and Communication
Shift Left Testing fosters an environment of enhanced collaboration and communication between developers, testers, and operations teams. This collaborative approach ensures that quality is a shared responsibility and not just relegated to testers at the end of the development pipeline.
Receiving immediate feedback on their code, enabling quick adjustments that align with both functional and quality requirements is a key factor for agile developers.
➡️Reduction in Development Costs and Time
By identifying and addressing defects early, Shift Left Testing approach significantly reduces the cost and time associated with fixing bugs in later stages of development or after release. The cost of fixing a bug after deployment can be exponentially higher than fixing it during the development phase.
It leads to more predictable development timelines, reduced pressure to fix issues under tight deadlines, and a decrease in the overall cost of development projects.
➡️Improved Product Quality and Customer Satisfaction
Shift Left Testing inherently leads to a better end product. With continuous testing and quality assurance from the start, the software is built on a foundation of quality, resulting in a more stable, performant, and secure application.
This not only enhances the reputation of the development team but also fosters a positive relationship with the end-users, who benefit from a superior product experience.
➡️Supports Agile and DevOps Practices
The Shift Left Testing approach is perfectly aligned with Agile and DevOps practices, which emphasize rapid development cycles, continuous integration (CI), and continuous delivery (CD).
For devs working in Agile environments, Shift Left Testing provides the framework for integrating testing into each sprint, ensuring that every iteration of the product is tested and validated. This seamless integration of testing into the CI/CD pipeline enables faster release cycles, with the confidence that each release maintains a high standard of quality.
Implementing Shift Left Testing Approach
Implementing Shift Left Testing in a development project involves a strategic shift in how testing is integrated into the software development lifecycle. This not only necessitates changes in processes and tools but also in the mindset of developers and testers.
Step 1: Integrate Testing into the Early Stages of Development
Example: Incorporate unit testing as part of the development process. Developers should write unit tests for their code before or alongside the development of the features.
# Example of a simple Python unit test for a function add(a, b)
import unittest
def add(a, b):
return a + b
class TestAddFunction(unittest.TestCase):
def test_add(self):
self.assertEqual(add(2, 3), 5)
self.assertEqual(add(-1, 1), 0)
self.assertEqual(add(-1, -1), -2)
if __name__ == '__main__':
unittest.main()
Step 2: Leverage Automation for Continuous Testing
Automate your testing processes to run tests continuously as code is integrated into the main branch. This involves setting up CI pipelines that automatically trigger tests upon code commits.
Example: Configure a CI pipeline using Jenkins, GitHub Actions, or GitLab CI to run your automated tests whenever new code is pushed to the repository.
# Example of a basic GitHub Actions workflow to run Python unit tests
name: Python Unit Tests
on: [push]
jobs:
build:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python
uses: actions/setup-python@v2
with:
python-version: '3.8'
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install -r requirements.txt
- name: Run tests
run: |
python -m unittest discover -s tests
Step 3: Foster a Culture of Quality and Collaboration
Shift Left Testing requires a cultural shift where quality is everyone's responsibility. Encourage developers, testers, and operations teams to collaborate closely from the project's inception.
Example: Implement pair programming sessions between developers and testers to discuss test strategies for new features. Use communication tools like Slack or Microsoft Teams to facilitate continuous dialogue around testing and quality.
Step 4: Implement Test-Driven Development (TDD)
TDD is a key practice in Shift Left Testing, where you write tests for a new feature before writing the code itself.
// Example of TDD for a simple JavaScript function to check if a number is even
// Step 1: Write the test
function isEven(num) {
// Test function will be implemented here
}
describe("isEven", function() {
it("returns true if the number is even", function() {
assert.equal(isEven(4), true);
});
it("returns false if the number is odd", function() {
assert.equal(isEven(5), false);
});
});
// Step 2: Implement the function to pass the test
function isEven(num) {
return num % 2 === 0;
}
Step 5: Embrace Feedback and Iteration
Use feedback from testing to continuously improve the software. Implement tools and practices that make it easy to report, track, and address issues discovered during testing.
Example: Utilize issue tracking systems like Jira or GitHub Issues to manage feedback from tests, ensuring that all stakeholders can view the status of issues and contribute to their resolution.
Best Practices and Techniques for Shift Left Testing
Adopting Shift Left Testing in software development projects involves more than just an early start to testing; it encompasses a set of best practices and techniques designed to optimize the process. These practices ensure that testing is not only proactive but also integrated seamlessly into the development lifecycle, contributing to the overall quality and efficiency of the project. Here are key best practices and techniques that agile teams should consider:
1. Embed Quality Assurance in Every Phase
Quality assurance (QA) should be a continuous concern, starting from the initial stages of development. This means integrating QA processes and considerations into the planning, design, coding, and deployment phases. It's crucial to establish quality criteria and testing goals early on, ensuring that each feature developed meets the predefined standards before moving forward.
2. Leverage Automation Wisely
While manual testing remains valuable for certain types of tests (e.g., exploratory testing), automation is a cornerstone of effective Shift Left Testing. Automate repetitive and time-consuming tests, such as regression tests, to save time and ensure consistency.
However, be selective in what you automate to avoid maintaining a cumbersome suite of tests that might become obsolete quickly. Focus on automating tests that provide the most value and are likely to be reused.
// Example: Automating a simple login test with Selenium WebDriver in JavaScript
const {Builder, By, Key, until} = require('selenium-webdriver');
async function exampleTest() {
let driver = await new Builder().forBrowser('firefox').build();
try {
await driver.get('<http://www.example.com/login>');
await driver.findElement(By.id('username')).sendKeys('testUser');
await driver.findElement(By.id('password')).sendKeys('testPassword', Key.RETURN);
await driver.wait(until.titleIs('Dashboard'), 1000);
} finally {
await driver.quit();
}
}
exampleTest();
3. Practice Test-Driven Development (TDD)
TDD is a powerful technique in Shift Left Testing, where developers write tests before writing the code that implements the functionality. This approach ensures that development is guided by tests, leading to code that is inherently more testable and aligned with the specified requirements.
4. Prioritize CI/CD
CI/CD practices are integral to Shift Left Testing, enabling continuous testing, integration, and deployment of code changes. Implement a CI/CD pipeline that automatically runs your test suite against every commit to the codebase, ensuring immediate feedback on the impact of changes.
5. Foster a Collaborative Culture
Shift Left Testing requires a culture of collaboration between developers, testers, and other stakeholders. Encourage open communication and shared responsibility for quality, breaking down the silos that traditionally separate development and testing teams. Tools like pair programming and code reviews can facilitate this collaboration, allowing developers and testers to share insights and knowledge.
6. Optimize Test Environments
Ensure that your testing environments closely mimic the production environment to uncover issues that could affect users. Use containerization tools like Docker to create consistent, easily replicable testing environments that can be spun up or down as needed.
7. Embrace Feedback Loops
Implement short, iterative development cycles that incorporate feedback from testing early and often. Use the results from each testing phase to refine and improve both the product and the testing process itself.
Challenges and Solutions
Implementing Shift Left Testing offers numerous benefits, including early detection of defects, improved code quality, and a more efficient development process. However, organizations may face several challenges while adopting this approach. Recognizing these challenges and identifying effective solutions is crucial for a smooth transition to Shift Left Testing.
Challenges
Cultural Resistance: Shifting testing left requires a cultural shift within the organization, moving away from traditional development and testing silos. Developers, testers, and operations teams must collaborate closely, which can be a significant change for teams used to working independently.
Skill Gaps: As testing moves earlier into the development cycle, developers may need to acquire new testing skills, and testers may need to gain a deeper understanding of the code. This skill gap can hinder the effective implementation of Shift Left Testing.
Integration Complexity: Incorporating testing into the early stages of development and ensuring continuous integration and delivery (CI/CD) can be technically complex. Setting up automated testing frameworks and CI/CD pipelines requires careful planning and execution.
Maintaining Test Quality and Coverage: As the amount of testing increases, maintaining high-quality and comprehensive test coverage becomes challenging. Ensuring that tests are effective and cover the necessary aspects of the code without becoming redundant or obsolete is crucial.
HyperTest To Mitigate These Challenges

HyperTest using its CLI can integrate natively with any CI tool used for automated releases, and tests every new change or update in the application automatically with a new PR or commit as the trigger
When a PR is raised by the dev using GitHub, GitLab, Bitbucket or any other version control system, 2 things happen:
Their CI server would receive a new event notification which would then let it build and deploy the app.
The native CLI utility of HyperTest is in parallel notified of these events, making it automatically run all the tests.
The best part is that the final report that HyperTest generates can be viewed by devs inside their VCS, without ever moving out.
Implementing Shift Left Testing, complemented by tools like HyperTest, allows teams to overcome the challenges associated with this approach. It enables a smoother transition to a testing paradigm that places quality at the forefront of software development, ensuring that applications are not only built right but also built to last.
For more insights into how HyperTest simplifies microservices testing, visit the website.
Use Case in FinTech Industry
Background
XYZ Corporation, a leading software development firm specializing in financial services applications, faced increasing pressure to accelerate its product development lifecycle while maintaining high standards of quality and security.
With the growing complexity of their applications and the need for faster release cycles, the traditional approach to testing was becoming a bottleneck, leading to delays in releases and increased costs associated with late-stage defect resolution.
Challenge
The primary challenge for XYZ Corporation was:
Reducing the time-to-market for new features and
Updates while ensuring that the software remained secure, reliable, and user-friendly.
The late discovery of bugs in the development cycle was leading to costly fixes, delayed product launches, and a negative impact on customer satisfaction.
Implementation of Shift Left Testing
To address these challenges, XYZ Corporation decided to invest in a Shift Left Testing approach. The initiative involved several key steps:
Integration of Automated Testing Tools: XYZ Corporation integrated automated testing tools into their CI/CD pipelines, enabling tests to be run automatically with every code commit.
Adoption of TDD: Developers were encouraged to adopt TDD practices, writing tests before writing the actual code to ensure that all new features met the testing criteria from the outset.
Enhanced Collaboration between Developers and Testers: The company fostered a culture of collaboration between the development and testing teams.
Results
The implementation of Shift Left Testing led to significant improvements for XYZ Corporation:
Reduced Time-to-Market: The time required to release new features and updates was reduced by 30%, thanks to early bug detection and resolution.
Improved Product Quality: The number of critical defects found after release decreased by 50%, resulting in higher customer satisfaction and reduced support costs.
Increased Efficiency: The development team reported a 20% increase in efficiency, as early testing reduced the need for extensive rework.
Conclusion
Quick fixes, big savings!
The adoption of Shift Left Testing represents a pivotal transformation in the software development lifecycle, offering a proactive approach to quality assurance that brings significant benefits to both the development process and the final product. By integrating testing early and throughout the development cycle, organizations can achieve faster release times, enhanced product quality, reduced costs, and improved team collaboration and morale.

In conclusion, Shift Left Testing is not merely a trend but a fundamental shift towards embedding quality deeply and consistently into software development. It offers a pathway to building software that not only meets but exceeds the demands of today's fast-paced, quality-conscious market. For organizations aiming to stay competitive and innovative, embracing Shift Left Testing is not just an option but a necessity.
Related to Integration Testing