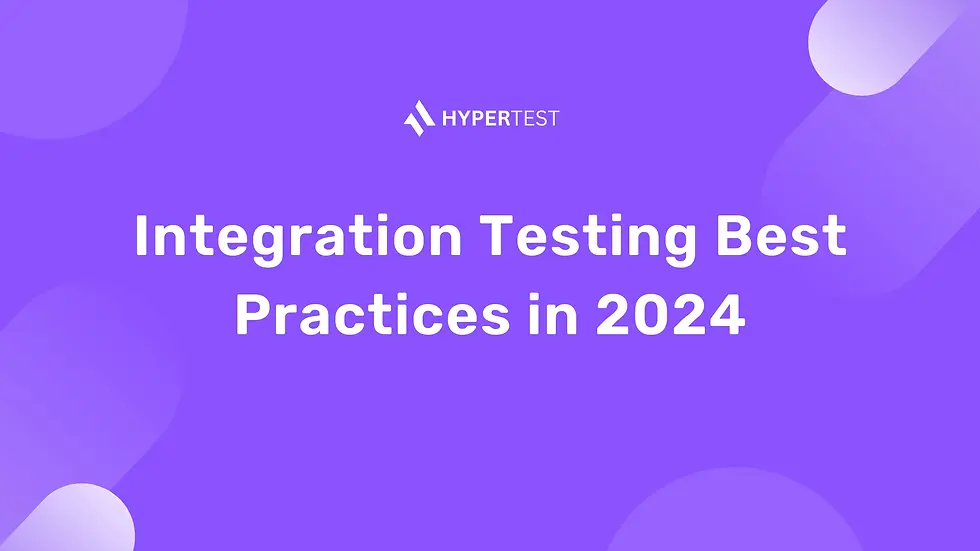
Fast Facts
Get a quick overview of this blog
Conduct integration tests in an environment that closely mirrors the production environment.
Try to mock or use stubs when testing integrations with external services.
Automation of integration tests can significantly improve efficiency and reliability.
Ensure that the integration tests cover all the critical interactions between different components of your application.
In the dynamic world of software development, integration testing stands as a critical phase, bridging the gap between unit testing and system testing. This process involves combining individual software modules and testing them as a group, ensuring they function seamlessly together.
The significance of integration testing lies in its ability to identify issues in the interaction between integrated units, which might not be evident in unit testing.
By catching these defects early, integration testing saves time and resources, and boosts the overall quality of the final product. This blog aims to delve into the best practices of integration testing, providing insights into its efficient implementation.
What is Integration Testing?
Integration testing is a level of software testing where individual units are combined and tested as a group. The primary goal is to expose faults in the interaction between integrated units.
Unlike unit testing, which focuses on individual components, or system testing, which evaluates the entire system, integration testing specifically targets the interfaces and communication between modules. This process is crucial in verifying the functional, performance, and reliability requirements of the software.
Importance of Integration Testing
Integration testing plays a pivotal role in software development. It ensures that software components, developed independently or in parallel, work together harmonically.
This testing phase is crucial for detecting interface defects, which might not be visible in unit testing. It verifies not only the functionality but also the communication and data transfer processes among modules.
By doing so, it helps maintain system integrity and consistency. Integration testing also validates performance and reliability requirements, offering insights into system behavior under various conditions.
Ultimately, it enhances software quality, reduces maintenance costs, and ensures a smoother, more reliable user experience.
Integration Testing Best Practices
Start Early and Test Often:
Incorporating integration testing early in the development cycle is vital. It enables the early detection of defects and reduces the cost of fixing them. Regular testing as new modules are integrated ensures continuous oversight.
Example: Consider a software project developing a web application. Starting integration tests when the first two modules (like user authentication and data retrieval) are developed can help identify any discrepancies or integration issues early on.
Choose the Right Tools:
Selecting appropriate tools is critical for effective integration testing. Tools should be compatible with the project's technology stack and support automation to streamline the testing process.

Example: For a project based on the Java ecosystem, tools like JUnit for unit testing and Selenium for web interface testing might be appropriate.
Feature / Tool | HyperTest | Katalon | JUnit | Postman |
Primary Use | Automated Integration Testing | Automated UI and API Testing | Unit Testing | API Testing |
Language Support | Language Agnostic | Groovy, Java | Java | JavaScript |
Platform | Cloud-based | Desktop, Cloud | Desktop | Desktop, Cloud |
Testing Type | Integration, System | Integration, E2E, Unit | Unit, Integration | Integration, API |
Target Audience | DevOps, Developers | QA Teams, Developers | Developers | Developers, QA Teams |
Automation | High level of automation | High | Moderate | High |
Ease of Setup | Easy | Moderate | Easy | Complex |
Integration | CI/CD pipelines, Various tools supported | CI/CD pipelines, JIRA, qTest | Various IDEs, Build tools | CI/CD pipelines, Various tools |
Cost | Varies (Free trial available) | Paid versions | Free | Paid versions |
Community & Support | Growing community, Professional support | Large community, Good support | Very large community, Extensive documentation | Large community, Extensive documentation |
Create a Test Plan:
A comprehensive test plan outlines the scope, approach, resources, and schedule of the testing activities. It should include specific test cases that cover all pathways and interactions between modules.
Example: A test plan for an e-commerce application might include test cases for user registration, product search, shopping cart functionality, and payment processing.
Test Environment Configuration:
Setting up a test environment that closely mirrors the production environment is crucial for accurate results. This includes configuring hardware, software, network settings, and other system components.
# Sample code to set up a test environment configuration
from test_environment_setup import configure_environment
# Configure settings for test environment
settings = {
"database": "test_db",
"server": "test_server",
"network_config": "VPN",
# More settings...
}
# Apply configuration
configure_environment(settings)
Continuous Integration: Implementing continuous integration practices allows for regular merging of code changes into a central repository, followed by automated builds and tests. This approach helps in identifying and addressing integration issues promptly.
Example: Using a tool like Jenkins or HyperTest to automatically run integration tests whenever new code is pushed to the repository.
Error Handling:
Effective integration testing should also focus on how the system handles errors. Testing should include scenarios where modules fail to communicate or return unexpected results.
# Example of a test case for error handling
def test_module_communication_failure():
response = module_a.communicate_with_module_b()
assert response.status_code == 500 # Expecting a failure status code
Performance Testing:
Explanation:
Besides checking functionality, it’s important to test the performance of integrated units under various load conditions.
Example: Conducting stress tests to evaluate how the system performs under high traffic or data load.
Documentation and Reporting:
Explanation: Maintaining detailed documentation of the testing process, including test cases, results, and issues, is essential for future reference and accountability. Regular reporting keeps all stakeholders informed about the testing progress and outcomes.
Example: Creating a shared online dashboard that updates in real-time with the status of ongoing integration tests and results.
Scenario: Adding a Product to the Shopping Cart
Overview
This test scenario will verify if the application correctly adds a product to the shopping cart, updating the cart's contents and the total price accordingly. It involves the integration of the product catalog, shopping cart, and pricing modules.
Components Involved
Product Catalog Module: Displays products.
Shopping Cart Module: Manages items in the cart.
Pricing Module: Calculates prices and totals.
Test Steps
Select a Product: The user navigates the product catalog and selects a product to purchase.
Add Product to Cart: The selected product is added to the shopping cart.
Verify Cart Contents: The cart should now include the new item.
Check Updated Price: The total price in the cart should update to reflect the addition of the new item.
Expected Results
The selected product should appear in the shopping cart.
The total price in the shopping cart should accurately reflect the addition of the new product.
Code for Integration Test
Here's a simplified example in Python using a hypothetical testing framework. This is for illustration purposes and assumes the presence of certain methods and classes to interact with the application.
import unittest
from app import ShoppingCart, ProductCatalog, PricingService
class TestAddToCart(unittest.TestCase):
def setUp(self):
self.cart = ShoppingCart()
self.catalog = ProductCatalog()
self.pricing = PricingService()
def test_add_product_to_cart(self):
# Step 1: Select a Product
product = self.catalog.select_product("ProductID_123")
# Step 2: Add Product to Cart
self.cart.add(product)
# Step 3: Verify Cart Contents
self.assertIn(product, self.cart.get_items(), "Product not in cart")
# Step 4: Check Updated Price
total_price = self.pricing.calculate_total(self.cart.get_items())
self.assertEqual(total_price, self.cart.get_total(), "Incorrect total price")
if __name__ == "__main__":
unittest.main()
Integration tests can be more complex depending on the application's size and the interactions between different modules. It's crucial to cover various scenarios, including edge cases, to ensure the robustness of the application.
Conclusion
Integration testing is an indispensable part of software development that ensures different modules of a software system work together flawlessly.
By adhering to the best practices outlined, such as early testing, choosing the right tools, and maintaining thorough documentation, teams can effectively mitigate risks and enhance the quality of their software products.
Remember, successful integration testing not only improves functionality but also contributes to a more reliable and robust software system.
Related to Integration Testing