20 November 2023
09 Min. Read
10 API Testing Tips for Beginners (SOAP & REST)
What is an API?
Imagine you have two apps on your phone - one for weather updates and another for your calendar. Now, you really want to see the weather forecast for the week right in your calendar app, without having to open the weather app separately. How do they communicate? This is where APIs come in.
An API (Application Programming Interface) is like a set of rules that allows different software applications to talk to each other. It's a way for one program to ask for information or services from another.
In our example, the weather app might have an API that lets it share weather data with the calendar app. This way, the calendar app can display the weather without having to know all the nitty-gritty details of how the weather app works.
So, APIs make it possible for different programs to work together, even if they are made by different developers or companies. It's like a language they all understand, allowing them to share and use each other's features seamlessly.
APIs serve as the connective tissue in modern software, enabling different systems and applications to communicate and exchange data seamlessly. They act as intermediaries, allowing different software programs to interact without needing to understand each other's underlying code.
What is API Testing?
API testing, at its core, aims to unveil inconsistencies and deviations, ensuring software functions as expected. Continuous testing is crucial, especially with public access, as the risk of releasing a flawed or insecure product outweighs testing costs.
APIs contribute value to an application, enhancing the intelligence of devices like phones and optimizing business workflows. A malfunctioning API, resulting from undetected errors, poses a risk not only to a single application but also to an entire interconnected chain of business processes.
Reasons to test your APIs:
Validate expected API performance
Ensure cross-compatibility across devices, browsers, and OS
Prevent costly repercussions in the future
Confirm capability to handle varying workloads
Investing additional effort in API testing contributes to a more robust end product. Ensuring that all data access, both read and write, exclusively occurs through the API streamlines security and compliance testing. This reinforces the overall integrity of the product.
Read more - What is API Testing? A Complete Guide
Why API Testing is important?
API testing is crucial in validating the functionality, reliability, performance, and security of these interfaces. Unlike traditional GUI testing, API testing focuses on the business logic layer of software architecture. It ensures that the APIs meet expectations and the integration between different systems functions as intended.
Ensuring Functionality: API testing verifies that different components of software communicate effectively, ensuring that the application functions as intended.
Early Detection of Bugs: By identifying issues early in the development process, API testing helps prevent the escalation of bugs and reduces the likelihood of costly fixes later on.
Cost-Efficiency: Detecting and fixing issues early in the development lifecycle through API testing is more cost-effective than addressing them in later stages or after deployment.
Enhanced Software Quality: Comprehensive API testing contributes to overall software quality by validating the performance, reliability, and security of the application.
Interoperability: API testing ensures that different software components can work together seamlessly, promoting interoperability and a smooth user experience.
Support for Continuous Integration/Continuous Deployment (CI/CD): Automated API testing aligns with CI/CD practices, enabling rapid and reliable software delivery through automated testing in the development pipeline.
Understanding API Types
This article focuses on providing beginners with practical tips for testing two predominant web service types: SOAP (Simple Object Access Protocol) and REST (Representational State Transfer).
So let’s understand these two types of API first, before diving straight into learning about the best practices for performing API testing.
SOAP vs. REST APIs
SOAP (Simple Object Access Protocol) and REST (REpresentational State Transfer) are two primary types of web services in API testing.
SOAP, defined by W3C standards, is a protocol for sending and receiving web service requests and responses, while REST is a more flexible, web standards-based architecture that uses HTTP without an official standard for APIs.
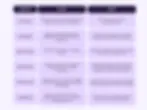
Understanding the nuances between these two API types is crucial for testers to apply the appropriate testing strategies.
How to test an API?
Testing an API is a critical step in software development to ensure functionality, reliability, and optimal performance.
Here's a simple guide on how to test an API effectively:
👉 Define Objectives: Identify API functionalities and testing scope.
👉 Plan Test Cases: Design cases for positive, negative, and boundary scenarios.
👉 Execute Tests: Utilize tools to send requests and validate responses.
👉 Assess Results: Analyze responses for accuracy, speed, and error handling.
👉 Report and Retest: Compile findings, share results, and retest after issue resolution. This systematic flow ensures thorough testing, validating API performance and functionality.
Now let’s take a overview of how to test the different types of APIs—the REST and the SOAP APIs.
Testing REST APIs:
Use of HTTP Methods: Test GET, POST, PUT, DELETE, PATCH methods for appropriate actions.
Stateless Operations: Ensure each request contains all necessary information and doesn't rely on server-stored context.
Response Codes: Verify correct use of HTTP status codes (200 for success, 404 for not found, etc.).
Data Formats: Test the API's ability to handle different data formats like JSON, XML, etc.
For REST API testing, the fetch API is commonly used in JavaScript. Here's an example for testing a REST API endpoint:
fetch('https://api.example.com/users/123')
.then(response => {
if (!response.ok) {
throw new Error('Network response was not ok ' + response.statusText);
}
return response.json();
})
.then(data => {
console.assert(data.user.id === 123, 'Incorrect User ID');
// Additional assertions as needed
})
.catch(error => {
console.error('There has been a problem with your fetch operation:', error);
});
This script sends a GET request to a REST API endpoint and includes basic error handling and an assertion to check the user ID in the response.
Testing SOAP APIs:
WSDL Document: Understand the structure defined in the WSDL (Web Services Description Language) document.
SOAP Envelope: Ensure the SOAP message is correctly structured with Header and Body tags.
Fault Handling: Test for proper SOAP fault generation in case of errors.
Security Testing: Check for WS-Security standards compliance and other security aspects like encryption and authentication.
Testing SOAP APIs in JavaScript is a bit more complex, as you need to send an XML request.
Now that we've covered the fundamentals, let's move on to examining the best practices, taking a closer look at each.
API Testing Best Practices
1. Comprehensive API Understanding
A deep comprehension of the API's intended functionality, data management, and integration points is paramount. This knowledge shapes the testing strategy, guiding the preparation of scenarios and validation of responses.
Before commencing testing, thoroughly review documentation and any available materials to establish a clear understanding of the API, ensuring anticipation of expected behavior and functionality.
2. Automate Where Possible
Embracing the intricacies of API testing demands a strategic perspective on streamlining processes. Automation plays a pivotal role in achieving efficiency.
By automating repetitive tasks, such as data management and verification processes, teams can execute tests more rapidly and consistently. This not only accelerates the testing lifecycle but also enhances the reliability of results.
Example: Overall Test Planning Automation
Without Automation:
# Manual test planning
execute_test_case("Scenario 1")
execute_test_case("Scenario 2")
With Automation:
# Automated test planning
execute_all_test_cases()
API testing, when treated as a fundamental aspect of software development, underscores its significance in ensuring the delivery of high-quality software. It aligns with modern development practices, contributing to robust and dependable software systems.
Make sure to invest in a codeless-automation tool like HyperTest, saving you time, efforts and money overtime.
3. Create Comprehensive Test Scenarios
Developing a diverse set of test scenarios, including both positive and negative cases, is vital for a comprehensive evaluation of the API. This approach guarantees that the API not only adeptly handles expected use cases but also gracefully manages erroneous or unexpected inputs, enhancing its overall resilience.
Example:
Positive Test Case: User Authentication
Scenario: Verify that the API correctly authenticates a user with valid credentials.
Example Code:
response = authenticate_user("valid_username", "secure_password")
assert response.status_code == 200
assert "access_token" in response.json()
Negative Test Case: Invalid Input Handling
Scenario: Assess how the API responds when provided with invalid or missing input.
Example Code:
response = authenticate_user("invalid_username", "weak_password")
assert response.status_code == 401
assert "error" in response.json()
By constructing a mix of scenarios like these, the testing process thoroughly evaluates the API's capabilities, ensuring it performs optimally under varying conditions and gracefully handles potential issues.
4. Use the Right API Testing Tools
The choice of tools can significantly impact the efficiency and effectiveness of the testing process. Factors like API type compatibility, ease of integration, and support for advanced testing features should guide the selection process.
While selecting an API automation tool, these must be the focus points:
It should include no or very little manual coding effort.
The tool should focus on end-to-end scenario testing, and should be self-sufficient.
It should save some time by automatically taking care of all the documentation and schemas.
It should be compatible with testing all major types of APIs i.e., GraphQL, gRPC, SOAP and REST.
Based on all these key points, we’ve already created a list of top 10 best performing API testing tools
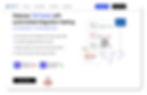
5. Run your tests in parallel for speed
Executing API tests in parallel is a powerful strategy to enhance testing speed and efficiency. Instead of running tests sequentially, parallel execution allows multiple tests to run simultaneously.
This not only accelerates the testing process but also identifies bottlenecks and scalability issues in the API more effectively.
Faster Execution
Scalability Assessment
Identifying Resource Limitations
Example: Consider an API with multiple endpoints. Running tests in parallel can involve executing tests for different endpoints concurrently:
$ pytest test_endpoint_1.py & pytest test_endpoint_2.py & pytest test_endpoint_3.py
This approach significantly reduces the overall testing time, making it a valuable strategy for teams working on continuous integration and deployment pipelines. However, ensure that your testing environment and infrastructure can support parallel execution effectively.
6. API testing should be a part of your CI/CD pipeline
Incorporating API testing into your CI/CD pipeline is a fundamental practice for maintaining a high standard of software quality throughout the development lifecycle.
This integration ensures that API tests are automatically executed whenever there's a change in the codebase, allowing teams to catch issues early and deliver reliable software consistently.
Automated Triggering of Tests
Early Detection of Issues
Consistency Across Environments
7. Keep your testing as simple as possible
Maintaining simplicity in API testing is a fundamental principle that can significantly enhance the efficiency and effectiveness of the testing process.
While it may be tempting to introduce complexity, especially with intricate APIs, simplicity often leads to clearer test cases, easier maintenance, and quicker identification of issues.
Guidelines for Simplifying API Testing:
Feature | Complex Approach | Simple Approach |
Focused Test Objectives | Testing multiple functionalities in a single test case | Break down test cases to focus on specific functionalities |
Clear Verification Points | Elaborate verification steps with unnecessary details | Clearly defined and focus on essential verification points |
Minimal Dependencies | Intertwining tests with dependencies on external factors | Minimize dependencies to ensure tests remain independent and reproducible |
8. Decipher the API Output Status
One of the fundamental aspects of effective API testing is mastering the interpretation of API response codes.
These status codes, included in the response from the server, convey crucial information about the success or failure of a request. Decoding these codes helps to identify issues and understand about APIs behavior in various scenarios.
👉 Immediate Feedback
The "200 OK" status code indicates a successful request. Understanding this code is crucial for confirming that the API processed the request as expected.
HTTP/1.1 200 OK
Content-Type: application/json
{
"status": "success",
"data": { ... }
}
👉 Error Identification
A "404 Not Found" status code signals that the requested resource is not available. It aids in promptly identifying and addressing issues.
HTTP/1.1 404 Not Found
Content-Type: application/json
{
"error": "Resource not found"
}
👉 Server-Side insights
The "500 Internal Server Error" code highlights a server-side issue. Understanding this code is vital for diagnosing and resolving problems affecting the server.
HTTP/1.1 500 Internal Server Error
Content-Type: application/json
{
"error": "Unexpected server error"
}
9. Test for failure
While validating positive scenarios is crucial, testing for failure is equally vital to enhance the resilience of your API. Negative testing involves deliberately subjecting the API to invalid inputs, unexpected conditions, or erroneous requests to assess how well it handles adverse situations.
Error Handling Evaluation
Testing scenarios with invalid inputs ensures that the API responds with clear error messages, guiding users or developers on how to rectify issues.
Boundary Testing
Assessing how the API handles requests with payloads beyond specified limits helps prevent potential data integrity or security issues.
Unexpected Input Handling
Testing for unexpected input formats ensures that the API gracefully handles variations, enhancing its versatility.
By incorporating negative test cases, you fortify your API against potential vulnerabilities, ensuring it behaves robustly under challenging conditions. This proactive testing approach contributes to the overall reliability and user satisfaction with your API.
10. Choosing Verification Methods
Selecting appropriate verification methods is a critical aspect of API testing, influencing the accuracy and thoroughness of the testing process.
It involves determining the most suitable techniques to confirm that the API functions as expected, providing reliable results and ensuring the delivery of high-quality software.
Example:
Validating headers and metadata ensures that the API adheres to specified standards and provides additional context for request handling.
HTTP/1.1 201 Created
Content-Type: application/json
Location: /api/users/123
Conclusion
API testing is an essential skill in the software development world.
By starting with a solid understanding of the type of API you’re working with (SOAP or REST), thoroughly exploring the documentation, and carefully selecting your testing tools, you can ensure a comprehensive testing process.
Remember to balance manual and automated testing, focus on both positive and negative scenarios, and never underestimate the importance of security and performance testing.
As you grow more comfortable with API testing, you’ll find it’s a dynamic and rewarding field, constantly evolving with new technologies and methodologies. Happy testing!